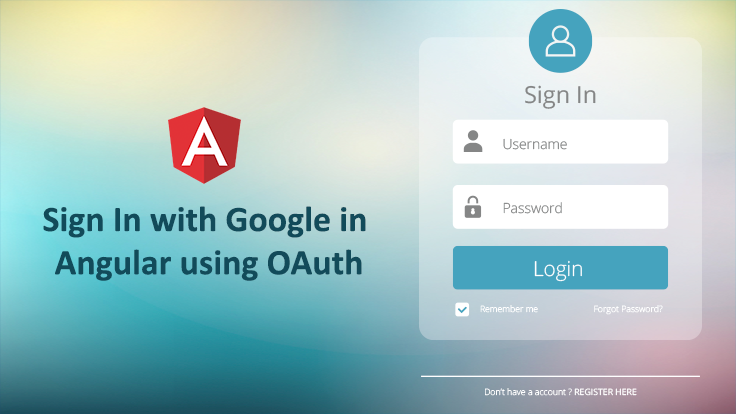
Angular is javascript’s most famous framework for building a single-page web application. There are many ready to use the library which enhances development speed and user experience but when we talk about user login, user login is not always easy to do and manage. So let’s have a look at the step-by-step process to user login into your angular web application with Google using OAuth.
Once a user gets login with google into our web app, you’ll get a confirmation that the user has valid email and profile on Google. Before we begin, let’s have an overview of OAuth and how does it work.
What is OAuth?
OAuth is a protocol that provides big website’s users’ information to any third party web app or mobile application without sharing the user’s password or any other personal information or any sensitive details.
Web giants websites like Google, Microsoft or Facebook allows its user to share his/her piece of information to any third party application or website while protecting sensitive and confidential information.
Security and Confidentiality
OAuth is a secure platform and maintain the confidentiality of the user’s data as it doesn’t share password data but uses auth tokens to prove an identity between consumers and service providers. OAuth allows interacting one application to service providers on behalf of the user without giving away the user’s password.
How does OAuth work?
When we first click on Sign Up with Google, it redirects you to www.gmail.com and checks whether you are already logged in or not. If you are not logged in then it prompts you to Login window with these fields- EmailId and Password. Once you’ve logged in, it will display a small dialogue box that shows what kind of information will be shared with this third party web app or mobile application. Now there’re 2 cases to move forward:
- If you agree to the information, then press the Continue button.
- If you are not okay with the information which is shared with the third-party web application you can easily edit your preference.
Now, Google redirects back you to a third party web application with an authentication code so that it’s clear that the user has authorized Google account or not.
The website can now show the unique code which was acquired while registering for the first time as a legal web application to Google and get the restricted users’ information consisting of your name, email address, and profile image, etc.
Why Login with Google?
- Reduces the burden of login for your users
- Gateway to connecting with Google’s users in a secure manner.
- Makes register or login process faster.
- Offers familiarity.
Generate ClientID
First, go to https://console.developers.google.com/ and create your project. Setup information about your project in OAuth consent screen, select the scope which might require your web app. Here the scope means user’s google information such as email id, profile picture.
After the consent setup, you have to create a client ID for your web app from the credentials section. We will also add localhost as authorized origins so that when we test, it will work.
Now select OAuth client ID from given options and provide necessary details for your web application and create client ID and copy client ID for further use.
Steps for Google login in Angular web application.
For logging with Google we have to install npm library “angular4-social-login” into our angular application.
npm install --save angular4-social-login
Imports these dependencies from angular4-social-login into your app.module.ts.
import { SocialLoginModule , AuthServiceConfig , GoogleLoginProvider } from 'angular4-social-login';
Copy Client ID which we had already created as above into your app.module.ts.
// client id for google login const google_auth_client_id : string = ".......................... Google Client ID…………………….."
Now configure client ID in object as below.
// configure client id in object let config = new AuthServiceConfig([{ id: GoogleLoginProvider.PROVIDER_ID, provider : new GoogleLoginProvider(google_auth_client_id) }])
Import SocialLoginModule as below
imports: [ ... SocialLoginModule.initialize(config) ],
For sign in and out users do the following. Create a constructor of the AuthService in your component.ts file.
Import the AuthService and GoogleLoginProvider from “angular4-social-login”.
Now, Create signIn() and signOut() method for login and logout functionality.
import { AuthService, GoogleLoginProvider} from 'angular4-social-login'; ... constructor(private _socioAuthServe : AuthService,private router : Router){}
Login function is as below,
//Method for sign in with google signIn(platform : string): void{ platform = GoogleLoginProvider.PROVIDER_ID; this._socioAuthServe.signIn(platform).then((response)=>{ this.user = response; } ); }
We receive a SocialUser object when the user logs in and a null when the user logs out. SocialUser object contains basic user information such as name, email, photo URL, etc.
Signout method for the same is as below.
//Method for sign in with google signOut() : void{ this._socioAuthServe.signOut(); }
We can display user’s information as below in our template file.
<img src="{{ user.photoUrl }}"> <div> <h4>{{ user.name }}</h4> <p>{{ user.email }}</p> </div>
Conclusion
Before wrapping up let’s go through a quick summary of what we’ve covered so far. So, OAuth basically gives third party application a special key that only provides restricted information about a user while maintaining security and Confidential details of users. We’ve learned the steps to generate client ID for Google login from console.developers.google.com, how to use client ID for login system in angular with help of integrating “angular4-social-login” library with the simple steps mentioned above.
Gone are the days when conventional approaches were used for doing business. Today, enterprise web application development can make the entire web portal more enticing. Looking for an intuitive, fast & robust web application? AIMDek serves as a full stack vendor undertaking end-to-end enterprise web application development projects for established market leaders as well as emerging businesses with technology at their heart. Bring on your queries at sales@aimdek.com